Cracking the Code: Google Search Console API Analysis Made Easy with Python
- 01 Jul, 2024
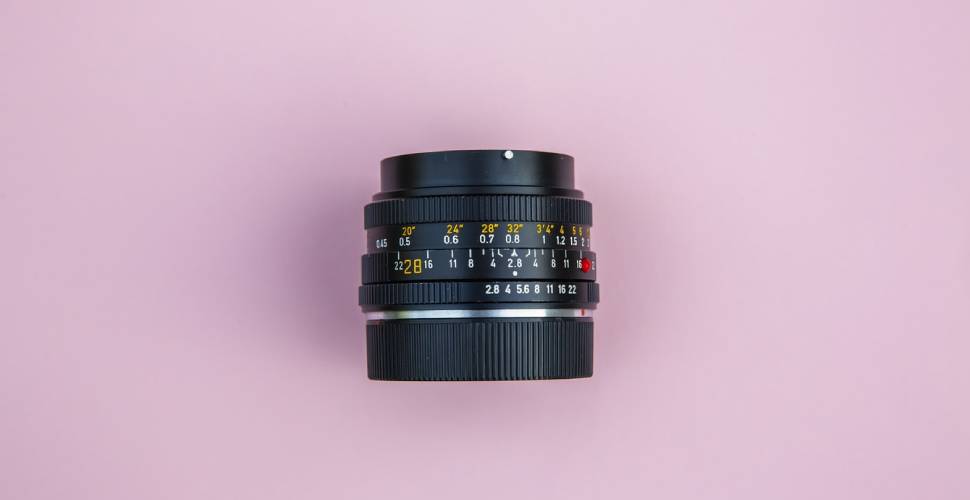
Cracking the Code: Google Search Console API Analysis Made Easy with Python
In the ever-evolving world of digital marketing, data is king. And when it comes to search performance data, Google Search Console (GSC) is a treasure trove. But what if you could go beyond the standard interface and dive deeper into your data? Enter the Google Search Console API and Python – a powerful combination that can unlock insights you never knew existed.
Why Use the Google Search Console API?
While the GSC web interface is user-friendly, it has limitations. The API allows you to:
- Access more data (up to 16 months of historical data)
- Combine GSC data with other data sources
- Automate your reporting process
- Perform custom analyses tailored to your specific needs
Setting Up Your Environment
Before we dive in, make sure you have the following:
- Python installed on your machine
- Google Search Console API credentials
- The
google-auth
andgoogle-auth-oauthlib
libraries installed
You can install the required libraries using pip:
pip install google-auth google-auth-oauthlib google-auth-httplib2 google-api-python-client
Authenticating with the API
First, let’s set up authentication:
from google.oauth2.credentials import Credentials
from googleapiclient.discovery import build
# Set up credentials (you'll need to have your OAuth 2.0 flow set up)
creds = Credentials.from_authorized_user_file('path/to/your/credentials.json')
# Build the service
service = build('searchconsole', 'v1', credentials=creds)
Fetching Data from the API
Now, let’s fetch some data:
import pandas as pd
from datetime import datetime, timedelta
# Set the parameters for your request
site_url = 'https://www.example.com/'
end_date = datetime.today().strftime('%Y-%m-%d')
start_date = (datetime.today() - timedelta(days=30)).strftime('%Y-%m-%d')
# Make the request
response = service.searchanalytics().query(
siteUrl=site_url,
body={
'startDate': start_date,
'endDate': end_date,
'dimensions': ['query', 'page'],
'rowLimit': 10000
}
).execute()
# Convert the response to a DataFrame
df = pd.DataFrame(response['rows'])
df.columns = ['query', 'page', 'clicks', 'impressions', 'ctr', 'position']
Analyzing the Data
With our data in a DataFrame, the possibilities are endless. Here are a few examples:
- Top Performing Pages
top_pages = df.groupby('page').sum().sort_values('clicks', ascending=False).head(10)
print(top_pages)
- Queries with High Impressions but Low CTR
low_ctr_queries = df[df['impressions'] > 1000].sort_values('ctr').head(10)
print(low_ctr_queries)
- Average Position by Query
avg_position = df.groupby('query')['position'].mean().sort_values().head(20)
print(avg_position)
Visualizing Your Data
Python’s data visualization libraries can help you create insightful charts. Here’s an example using matplotlib:
import matplotlib.pyplot as plt
plt.figure(figsize=(12,6))
plt.scatter(df['ctr'], df['position'], alpha=0.5)
plt.xlabel('CTR')
plt.ylabel('Average Position')
plt.title('CTR vs Average Position')
plt.show()
Automating Your Analysis
The real power comes from automation. You can schedule your script to run daily, weekly, or monthly, and automatically send reports to your team.
import schedule
import time
def job():
# Your analysis code here
# Send email with results
schedule.every().monday.at("09:00").do(job)
while True:
schedule.run_pending()
time.sleep(1)
Conclusion
The Google Search Console API, combined with Python’s data analysis capabilities, opens up a world of possibilities for content marketing professionals. By automating data collection and analysis, you can spend less time on repetitive tasks and more time on strategic decision-making.
Remember, the examples provided here are just the tip of the iceberg. As you become more comfortable with the API and Python, you’ll discover countless ways to slice and dice your data to gain valuable insights.
Happy coding, and may your digital marketing efforts be ever fruitful!